Autonomous authentication
Using autonomous authentication, neither your RSA private key used by your client id nor the password of the User ID are sent over the wire.
All requests are done over HTTPS using TLS 1.3.
Overview of the Autonomous authentication flow.
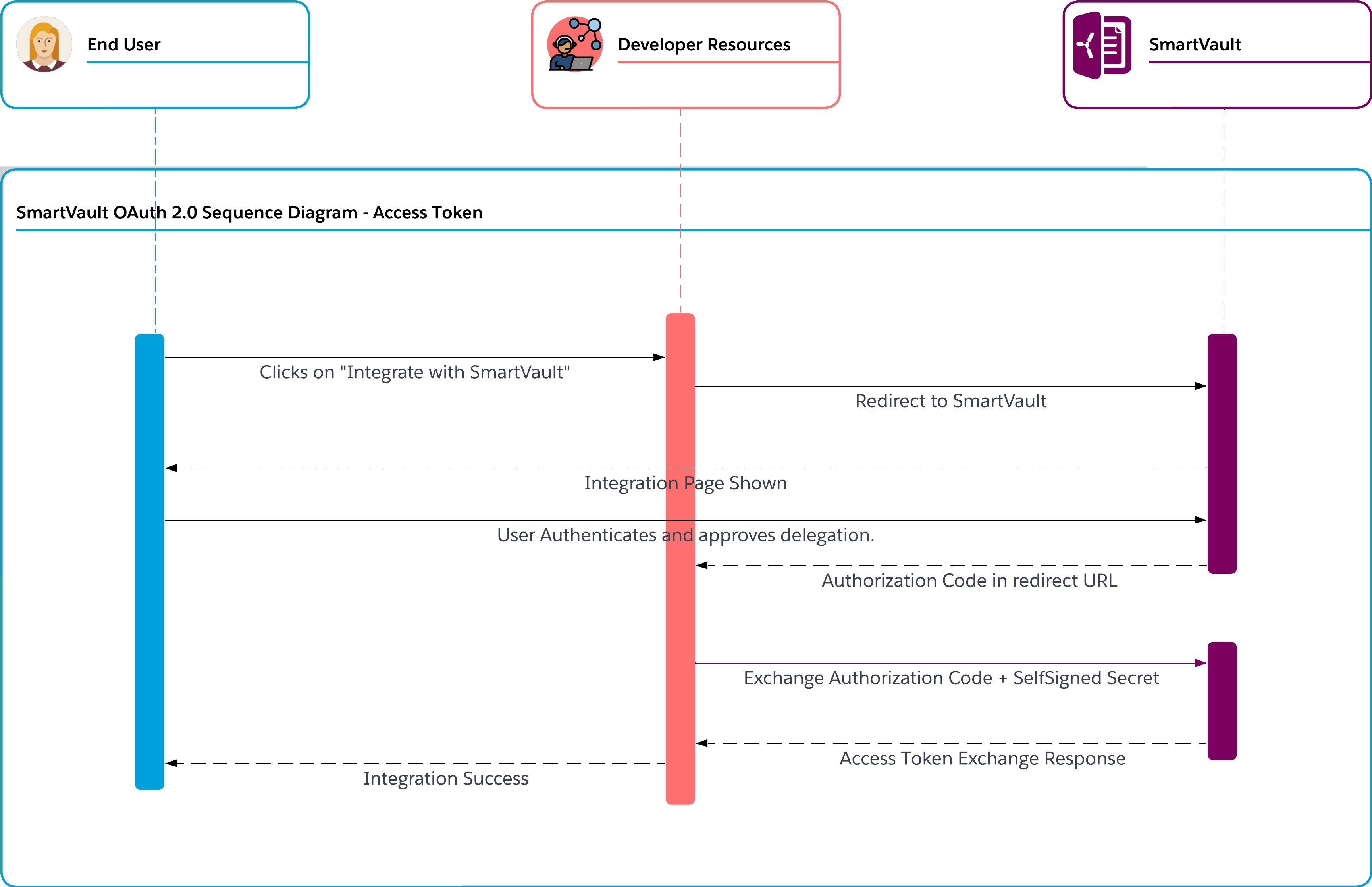
Request a nonce
A nonce is a string generated by the server that you will need in order to form a self-signed token. You'll need a client id for this.
More info on how to register a new client here.
Body parameters
client_id
stringThe client ID as registered in SmartVault.{"client_id": "SampleCRMWeb"}
Request
Headers:Content-Type:application/jsonAccept:application/jsonBody:{"client_id": "SampleCRMWeb"}
curl --include \--request POST \--header "Content-Type: application/json" \--header "Accept: application/json" \--data-binary "{ \"client_id\": \"SampleCRMWeb\" }" \'https://rest.smartvault.com/auto/auth/nonce/1'
// Maven : Add these dependecies to your pom.xml (java6+)// <dependency>// <groupId>org.glassfish.jersey.core</groupId>// <artifactId>jersey-client</artifactId>// <version>2.8</version>// </dependency>// <dependency>// <groupId>org.glassfish.jersey.media</groupId>// <artifactId>jersey-media-json-jackson</artifactId>// <version>2.8</version>// </dependency>import javax.ws.rs.client.Client;import javax.ws.rs.client.ClientBuilder;import javax.ws.rs.client.Entity;import javax.ws.rs.core.Response;import javax.ws.rs.core.MediaType;Client client = ClientBuilder.newClient();Entity payload = Entity.json("{ \"client_id\": \"SampleCRMWeb\"}");Response response = client.target("https://rest.smartvault.com/auto/auth/nonce/1").request(MediaType.APPLICATION_JSON_TYPE).header("Accept", "application/json").post(payload);System.out.println("status: " + response.getStatus());System.out.println("headers: " + response.getHeaders());System.out.println("body:" + response.readEntity(String.class));
var request = new XMLHttpRequest();request.open('POST', 'https://rest.smartvault.com/auto/auth/nonce/1');request.setRequestHeader('Content-Type', 'application/json');request.setRequestHeader('Accept', 'application/json');request.onreadystatechange = function () {if (this.readyState === 4) {console.log('Status:', this.status);console.log('Headers:', this.getAllResponseHeaders());console.log('Body:', this.responseText);}};var body = {'client_id': 'SampleCRMWeb'};request.send(JSON.stringify(body));
var request = require('request');request({method: 'POST',url: 'https://rest.smartvault.com/auto/auth/nonce/1',headers: {'Content-Type': 'application/json','Accept': 'application/json'},body: "{ \"client_id\": \"SampleCRMWeb\"}"}, function (error, response, body) {console.log('Status:', response.statusCode);console.log('Headers:', JSON.stringify(response.headers));console.log('Response:', body);});
$ENV{'PERL_LWP_SSL_VERIFY_HOSTNAME'} = 0;use LWP::UserAgent;use strict;use warnings;use 5.010;use Cpanel::JSON::XS qw(encode_json decode_json);my $ua = LWP::UserAgent->new;my $data = '{ "client_id": "SampleCRMWeb"}';$ua->default_header("Content-Type" => "application/json");$ua->default_header("Accept" => "application/json");my $response = $ua->post("https://rest.smartvault.com/auto/auth/nonce/1", Content => $data);print $response->as_string;
from urllib2 import Request, urlopenvalues = """{"client_id": "SampleCRMWeb"}"""headers = {'Content-Type': 'application/json','Accept': 'application/json'}request = Request('https://rest.smartvault.com/auto/auth/nonce/1', data=values, headers=headers)response_body = urlopen(request).read()print(response_body)
<?php$ch = curl_init();curl_setopt($ch, CURLOPT_URL, "https://rest.smartvault.com/auto/auth/nonce/1");curl_setopt($ch, CURLOPT_RETURNTRANSFER, TRUE);curl_setopt($ch, CURLOPT_HEADER, FALSE);curl_setopt($ch, CURLOPT_POST, TRUE);curl_setopt($ch, CURLOPT_POSTFIELDS, "{\"client_id\": \"SampleCRMWeb\"}");curl_setopt($ch, CURLOPT_HTTPHEADER, array("Content-Type: application/json","Accept: application/json"));$response = curl_exec($ch);curl_close($ch);var_dump($response);
require 'rubygems' if RUBY_VERSION < '1.9'require 'rest_client'values = '{"client_id": "SampleCRMWeb"}'headers = {:content_type => 'application/json',:accept => 'application/json'}response = RestClient.post 'https://rest.smartvault.com/auto/auth/nonce/1', values, headersputs response
package mainimport ("bytes""fmt""io/ioutil""net/http")func main() {client := &http.Client{}body := []byte("{\n \"client_id\": \"SampleCRMWeb\"\n}")req, _ := http.NewRequest("POST", "https://rest.smartvault.com/auto/auth/nonce/1", bytes.NewBuffer(body))req.Header.Add("Content-Type", "application/json")req.Header.Add("Accept", "application/json")resp, err := client.Do(req)if err != nil {fmt.Println("Errored when sending request to the server")return}defer resp.Body.Close()resp_body, _ := ioutil.ReadAll(resp.Body)fmt.Println(resp.Status)fmt.Println(string(resp_body))}
//Common testing requirement. If you are consuming an API in a sandbox/test region, uncomment this line of code ONLY for non production uses.//System.Net.ServicePointManager.ServerCertificateValidationCallback = delegate { return true; };//Be sure to run "Install-Package Microsoft.Net.Http" from your nuget command line.using System;using System.Net.Http;var baseAddress = new Uri("https://rest.smartvault.com/");using (var httpClient = new HttpClient{ BaseAddress = baseAddress }){httpClient.DefaultRequestHeaders.TryAddWithoutValidation("accept", "application/json");using (var content = new StringContent("{ \"client_id\": \"SampleCRMWeb\"}", System.Text.Encoding.Default, "application/json")){using (var response = await httpClient.PostAsync("auto/auth/nonce/1", content)){string responseData = await response.Content.ReadAsStringAsync();}}}
Dim request = TryCast(System.Net.WebRequest.Create("https://rest.smartvault.com/auto/auth/nonce/1"), System.Net.HttpWebRequest)request.Method = "POST"request.ContentType = "application/json"request.Accept = "application/json"Using writer = New System.IO.StreamWriter(request.GetRequestStream())Dim byteArray As Byte() = System.Text.Encoding.UTF8.GetBytes("{\""client_id\"": \""SampleCRMWeb\""}")request.ContentLength = byteArray.Lengthwriter.Write(byteArray)writer.Close()End UsingDim responseContent As StringUsing response = TryCast(request.GetResponse(), System.Net.HttpWebResponse)Using reader = New System.IO.StreamReader(response.GetResponseStream())responseContent = reader.ReadToEnd()End UsingEnd Using
import groovyx.net.http.RESTClientimport static groovyx.net.http.ContentType.JSONimport groovy.json.JsonSlurperimport groovy.json.JsonOutput@Grab (group = 'org.codehaus.groovy.modules.http-builder', module = 'http-builder', version = '0.5.0')def client = new RESTClient("https://rest.smartvault.com")def emptyHeaders = [:]emptyHeaders."Content-Type" = "application/json"emptyHeaders."Accept" = "application/json"def jsonObj = new JsonSlurper().parseText('{"client_id": "SampleCRMWeb"}')response = client.post( path : "/auto/auth/nonce/1",body : jsonObj,headers: emptyHeaders,contentType : JSON )println("Status:" + response.status)if (response.data) {println("Content Type: " + response.contentType)println("Body:\n" + JsonOutput.prettyPrint(JsonOutput.toJson(response.data)))}
NSURL *URL = [NSURL URLWithString:@"https://rest.smartvault.com/auto/auth/nonce/1"];NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:URL];[request setHTTPMethod:@"POST"];[request setValue:@"application/json" forHTTPHeaderField:@"Content-Type"];[request setValue:@"application/json" forHTTPHeaderField:@"Accept"];[request setHTTPBody:[@"{\n \"client_id\": \"SampleCRMWeb\"\n}" dataUsingEncoding:NSUTF8StringEncoding]];NSURLSession *session = [NSURLSession sharedSession];NSURLSessionDataTask *task = [session dataTaskWithRequest:requestcompletionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {if (error) {// Handle error...return;}if ([response isKindOfClass:[NSHTTPURLResponse class]]) {NSLog(@"Response HTTP Status code: %ld\n", (long)[(NSHTTPURLResponse *)response statusCode]);NSLog(@"Response HTTP Headers:\n%@\n", [(NSHTTPURLResponse *)response allHeaderFields]);}NSString* body = [[NSString alloc] initWithData:data encoding:NSUTF8StringEncoding];NSLog(@"Response Body:\n%@\n", body);}];[task resume];
import Foundation// NOTE: Uncommment following two lines for use in a Playground// import PlaygroundSupport// PlaygroundPage.current.needsIndefiniteExecution = truelet url = URL(string: "https://rest.smartvault.com/auto/auth/nonce/1")!var request = URLRequest(url: url)request.httpMethod = "POST"request.addValue("application/json", forHTTPHeaderField: "Content-Type")request.addValue("application/json", forHTTPHeaderField: "Accept")request.httpBody = """"{\n \"client_id\": \"SampleCRMWeb\"\n}"""".data(using: .utf8)let task = URLSession.shared.dataTask(with: request) { data, response, error inif let response = response {print(response)if let data = data, let body = String(data: data, encoding: .utf8) {print(body)}} else {print(error ?? "Unknown error")}}task.resume()
Response
Returns the nonce after the code property.
Show success object
Will return an error object if the client id is not specified in the request body.
Show error object
Request a client token
This call is used to request a client token. This is the token you will use to authenticate each time your application makes a call to the API.
The client token is used for administrative tasks like adding users and delegating on behalf of users.
In order to request a client token, you will need to form a self-signed token using the nonce and Client ID:
// Structure to generate a self-signed tokenBASE64("SLF00" + Length(UTF8(Client ID)) + UTF8(Client ID) + Length(Nonce) + NonceSIGN(SHA256("SLF00" + Length(UTF8(ClientId)) + UTF8(ClientId) + Length(Nonce) + Nonce))
Where the reserved names used correspond to:
Code snippets
Find below some snippets that may help you build the code needed for retrieving the client token.
Use of external dependencies may be being used in these code snippets. e.g. "BouncyCastle" is an OpenSource library for the C# example.Show code snippets
Body parameters
token
stringThe self-signed access token that you formed using the Client ID and the nonce.{"token": "U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw=="}
Request
Headers:Content-Type:application/jsonAccept:application/jsonBody:{"token": "U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw=="}
curl --include \--request POST \--header "Content-Type: application/json" \--header "Accept: application/json" \--data-binary "{ \"token\": \"U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw==\" }" \'https://rest.smartvault.com/auto/auth/ctoken/1'
// Maven : Add these dependecies to your pom.xml (java6+)// <dependency>// <groupId>org.glassfish.jersey.core</groupId>// <artifactId>jersey-client</artifactId>// <version>2.8</version>// </dependency>// <dependency>// <groupId>org.glassfish.jersey.media</groupId>// <artifactId>jersey-media-json-jackson</artifactId>// <version>2.8</version>// </dependency>import javax.ws.rs.client.Client;import javax.ws.rs.client.ClientBuilder;import javax.ws.rs.client.Entity;import javax.ws.rs.core.Response;import javax.ws.rs.core.MediaType;Client client = ClientBuilder.newClient();Entity payload = Entity.json("{ \"token\": \"U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw==\"}");Response response = client.target("https://rest.smartvault.com/auto/auth/ctoken/1").request(MediaType.APPLICATION_JSON_TYPE).header("Accept", "application/json").post(payload);System.out.println("status: " + response.getStatus());System.out.println("headers: " + response.getHeaders());System.out.println("body:" + response.readEntity(String.class));
var request = new XMLHttpRequest();request.open('POST', 'https://rest.smartvault.com/auto/auth/ctoken/1');request.setRequestHeader('Content-Type', 'application/json');request.setRequestHeader('Accept', 'application/json');request.onreadystatechange = function () {if (this.readyState === 4) {console.log('Status:', this.status);console.log('Headers:', this.getAllResponseHeaders());console.log('Body:', this.responseText);}};var body = {'token': 'U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw=='};request.send(JSON.stringify(body));
var request = require('request');request({method: 'POST',url: 'https://rest.smartvault.com/auto/auth/ctoken/1',headers: {'Content-Type': 'application/json','Accept': 'application/json'},body: "{ \"token\": \"U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw==\"}"}, function (error, response, body) {console.log('Status:', response.statusCode);console.log('Headers:', JSON.stringify(response.headers));console.log('Response:', body);});
$ENV{'PERL_LWP_SSL_VERIFY_HOSTNAME'} = 0;use LWP::UserAgent;use strict;use warnings;use 5.010;use Cpanel::JSON::XS qw(encode_json decode_json);my $ua = LWP::UserAgent->new;my $data = '{ "token": "U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw=="}';$ua->default_header("Content-Type" => "application/json");$ua->default_header("Accept" => "application/json");my $response = $ua->post("https://rest.smartvault.com/auto/auth/ctoken/1", Content => $data);print $response->as_string;
def get_unsigned_token(nonce: str, client_id: str) -> bytes:unsigned_token = "SLF00" + chr(len(client_id)) + client_id + chr(len(nonce)) + nonceunsigned_token = bytes(unsigned_token, "ascii")return unsigned_tokendef sign_token(private_key_string: str, unsigned_token: bytes) -> bytes:#must have the pycryptodome package installedfrom Crypto.PublicKey import RSAfrom Crypto.Signature.pkcs1_15 import PKCS115_SigSchemefrom Crypto.Hash import SHA256private_key = RSA.import_key(private_key_string)hashed_token = SHA256.new(unsigned_token)signer = PKCS115_SigScheme(private_key)signature = signer.sign(hashed_token)return signaturedef make_packet(unsigned_token: bytes, signature: bytes) -> str:import base64combined = unsigned_token + signatureself_signed_token = base64.b64encode(combined)self_signed_token = self_signed_token.decode('ascii')return self_signed_token#the main script starts hereimport requestsheaders = {'Content-Type': 'application/json','Accept': 'application/json'}private_key_string="""%YOUR PEM STRING%"""nonce = "%YOUR_NONCE%"client_id = "%YOUR_CLIENT_ID%"unsigned_token = get_unsigned_token(nonce=nonce,client_id=client_id)signed_token = sign_token(private_key_string=private_key_string,unsigned_token=unsigned_token)self_signed_token = make_packet(unsigned_token=unsigned_token,signature=signed_token)json = {}json["token"] = self_signed_tokenresponse = requests.post(url="https://rest.smartvault.com/auto/auth/ctoken/1",headers=headers,json=json)response_body = response.contentprint(response_body)
<?php$ch = curl_init();curl_setopt($ch, CURLOPT_URL, "https://rest.smartvault.com/auto/auth/ctoken/1");curl_setopt($ch, CURLOPT_RETURNTRANSFER, TRUE);curl_setopt($ch, CURLOPT_HEADER, FALSE);curl_setopt($ch, CURLOPT_POST, TRUE);curl_setopt($ch, CURLOPT_POSTFIELDS, "{\"token\": \"U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw==\"}");curl_setopt($ch, CURLOPT_HTTPHEADER, array("Content-Type: application/json","Accept: application/json"));$response = curl_exec($ch);curl_close($ch);var_dump($response);
require 'rubygems' if RUBY_VERSION < '1.9'require 'rest_client'values = '{"token": "U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw=="}'headers = {:content_type => 'application/json',:accept => 'application/json'}response = RestClient.post 'https://rest.smartvault.com/auto/auth/ctoken/1', values, headersputs response
package mainimport ("bytes""fmt""io/ioutil""net/http")func main() {client := &http.Client{}body := []byte("{\n \"token\": \"U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw==\"\n}")req, _ := http.NewRequest("POST", "https://rest.smartvault.com/auto/auth/ctoken/1", bytes.NewBuffer(body))req.Header.Add("Content-Type", "application/json")req.Header.Add("Accept", "application/json")resp, err := client.Do(req)if err != nil {fmt.Println("Errored when sending request to the server")return}defer resp.Body.Close()resp_body, _ := ioutil.ReadAll(resp.Body)fmt.Println(resp.Status)fmt.Println(string(resp_body))}
//Common testing requirement. If you are consuming an API in a sandbox/test region, uncomment this line of code ONLY for non production uses.//System.Net.ServicePointManager.ServerCertificateValidationCallback = delegate { return true; };//Be sure to run "Install-Package Microsoft.Net.Http" from your nuget command line.using System;using System.Net.Http;var baseAddress = new Uri("https://rest.smartvault.com/");using (var httpClient = new HttpClient{ BaseAddress = baseAddress }){httpClient.DefaultRequestHeaders.TryAddWithoutValidation("accept", "application/json");using (var content = new StringContent("{ \"token\": \"U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw==\"}", System.Text.Encoding.Default, "application/json")){using (var response = await httpClient.PostAsync("auto/auth/ctoken/1", content)){string responseData = await response.Content.ReadAsStringAsync();}}}
Dim request = TryCast(System.Net.WebRequest.Create("https://rest.smartvault.com/auto/auth/ctoken/1"), System.Net.HttpWebRequest)request.Method = "POST"request.ContentType = "application/json"request.Accept = "application/json"Using writer = New System.IO.StreamWriter(request.GetRequestStream())Dim byteArray As Byte() = System.Text.Encoding.UTF8.GetBytes("{\""token\"": \""U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw==\""}")request.ContentLength = byteArray.Lengthwriter.Write(byteArray)writer.Close()End UsingDim responseContent As StringUsing response = TryCast(request.GetResponse(), System.Net.HttpWebResponse)Using reader = New System.IO.StreamReader(response.GetResponseStream())responseContent = reader.ReadToEnd()End UsingEnd Using
import groovyx.net.http.RESTClientimport static groovyx.net.http.ContentType.JSONimport groovy.json.JsonSlurperimport groovy.json.JsonOutput@Grab (group = 'org.codehaus.groovy.modules.http-builder', module = 'http-builder', version = '0.5.0')def client = new RESTClient("https://rest.smartvault.com")def emptyHeaders = [:]emptyHeaders."Content-Type" = "application/json"emptyHeaders."Accept" = "application/json"def jsonObj = new JsonSlurper().parseText('{"token": "U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw=="}')response = client.post( path : "/auto/auth/ctoken/1",body : jsonObj,headers: emptyHeaders,contentType : JSON )println("Status:" + response.status)if (response.data) {println("Content Type: " + response.contentType)println("Body:\n" + JsonOutput.prettyPrint(JsonOutput.toJson(response.data)))}
NSURL *URL = [NSURL URLWithString:@"https://rest.smartvault.com/auto/auth/ctoken/1"];NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:URL];[request setHTTPMethod:@"POST"];[request setValue:@"application/json" forHTTPHeaderField:@"Content-Type"];[request setValue:@"application/json" forHTTPHeaderField:@"Accept"];[request setHTTPBody:[@"{\n \"token\": \"U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw==\"\n}" dataUsingEncoding:NSUTF8StringEncoding]];NSURLSession *session = [NSURLSession sharedSession];NSURLSessionDataTask *task = [session dataTaskWithRequest:requestcompletionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {if (error) {// Handle error...return;}if ([response isKindOfClass:[NSHTTPURLResponse class]]) {NSLog(@"Response HTTP Status code: %ld\n", (long)[(NSHTTPURLResponse *)response statusCode]);NSLog(@"Response HTTP Headers:\n%@\n", [(NSHTTPURLResponse *)response allHeaderFields]);}NSString* body = [[NSString alloc] initWithData:data encoding:NSUTF8StringEncoding];NSLog(@"Response Body:\n%@\n", body);}];[task resume];
import Foundation// NOTE: Uncommment following two lines for use in a Playground// import PlaygroundSupport// PlaygroundPage.current.needsIndefiniteExecution = truelet url = URL(string: "https://rest.smartvault.com/auto/auth/ctoken/1")!var request = URLRequest(url: url)request.httpMethod = "POST"request.addValue("application/json", forHTTPHeaderField: "Content-Type")request.addValue("application/json", forHTTPHeaderField: "Accept")request.httpBody = """"{\n \"token\": \"U0xGMDAMU2FtcGxlQ1JNV2ViFmNmRFh1bkhDYTBXZW5tUVhuU3BJOUHEXtJ+Je5g/igf0DtUcPmPw/5MPyxzZxzrKksa8UObxuiOOtFg38hL3cEMs67ggPwPZGwVF4WMb2Ix+7xGtfp0WPBRzkwUQMJZKGmBJ5PRFkGmX5M4vjmLriwFjYXa0xsGPArgQa2/dPW2gKt0xx1nAQbntDjH7kkbxoKxO+Rklw==\"\n}"""".data(using: .utf8)let task = URLSession.shared.dataTask(with: request) { data, response, error inif let response = response {print(response)if let data = data, let body = String(data: data, encoding: .utf8) {print(body)}} else {print(error ?? "Unknown error")}}task.resume()
Response
Returns the client token and its expiracy.
Show success object
Will return an error object if the token built is not specified in the request body or not valid.
Request a delegation token
The delegation token is used to perform actions on a user's behalf.
The user must have authorized your application in order for you to be able to do this. If not authorized, this call will return an access denied error.
To perform this authorization process, check here.
If you are using autonomous authentication, you will need to use the "Authorization" header to authenticate this request using the client id and the client token that you should have retrieved earlier.
The basic access authorization header value for this request needs to be:
clientId + ':' + clientToken
For the "user_email" body parameter, you need to specify your SmartVault account email, not the one you used to request the delegation token (the developer account email).
Body parameters
user_email
stringThe SmartVault account email address (not the developer one!).{"user_email": "test@smartvault.com"}
Request
Headers:Content-Type:application/jsonAccept:application/jsonAuthorization: Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==Body:{"user_email": "test@smartvault.com"}
curl --include \--request POST \--header "Content-Type: application/json" \--header "Accept: application/json" \--header "Authorization: Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==" \--data-binary "{\"user_email\": \"test@smartvault.com\"}" \'https://rest.smartvault.com/auto/auth/dtoken/1'
// Maven : Add these dependecies to your pom.xml (java6+)// <dependency>// <groupId>org.glassfish.jersey.core</groupId>// <artifactId>jersey-client</artifactId>// <version>2.8</version>// </dependency>// <dependency>// <groupId>org.glassfish.jersey.media</groupId>// <artifactId>jersey-media-json-jackson</artifactId>// <version>2.8</version>// </dependency>import javax.ws.rs.client.Client;import javax.ws.rs.client.ClientBuilder;import javax.ws.rs.client.Entity;import javax.ws.rs.core.Response;import javax.ws.rs.core.MediaType;Client client = ClientBuilder.newClient();Entity payload = Entity.json("{ \"user_email\": \"test@smartvault.com\"}");Response response = client.target("https://rest.smartvault.com/auto/auth/dtoken/1").request(MediaType.APPLICATION_JSON_TYPE).header("Authorization", "Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==").header("Accept", "application/json").post(payload);System.out.println("status: " + response.getStatus());System.out.println("headers: " + response.getHeaders());System.out.println("body:" + response.readEntity(String.class));
var request = new XMLHttpRequest();request.open('POST', 'https://rest.smartvault.com/auto/auth/dtoken/1');request.setRequestHeader('Authorization', 'Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==');request.setRequestHeader('Content-Type', 'application/json');request.setRequestHeader('Accept', 'application/json');request.onreadystatechange = function () {if (this.readyState === 4) {console.log('Status:', this.status);console.log('Headers:', this.getAllResponseHeaders());console.log('Body:', this.responseText);}};var body = {'user_email': 'test@smartvault.com'};request.send(JSON.stringify(body));
var request = require('request');request({method: 'POST',url: 'https://rest.smartvault.com/auto/auth/dtoken/1',headers: {'Authorization': 'Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==','Content-Type': 'application/json','Accept': 'application/json'},body: "{ \"user_email\": \"test@smartvault.com\"}"}, function (error, response, body) {console.log('Status:', response.statusCode);console.log('Headers:', JSON.stringify(response.headers));console.log('Response:', body);});
$ENV{'PERL_LWP_SSL_VERIFY_HOSTNAME'} = 0;use LWP::UserAgent;use strict;use warnings;use 5.010;use Cpanel::JSON::XS qw(encode_json decode_json);my $ua = LWP::UserAgent->new;my $data = '{ "user_email": "test@smartvault.com"}';$ua->default_header("Authorization" => "Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==");$ua->default_header("Content-Type" => "application/json");$ua->default_header("Accept" => "application/json");my $response = $ua->post("https://rest.smartvault.com/auto/auth/dtoken/1", Content => $data);print $response->as_string;
from urllib2 import Request, urlopenvalues = """{"user_email": "test@smartvault.com"}"""headers = {'Authorization': 'Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==','Content-Type': 'application/json','Accept': 'application/json'}request = Request('https://rest.smartvault.com/auto/auth/dtoken/1', data=values, headers=headers)response_body = urlopen(request).read()print response_body
<?php$ch = curl_init();curl_setopt($ch, CURLOPT_URL, "https://rest.smartvault.com/auto/auth/dtoken/1");curl_setopt($ch, CURLOPT_RETURNTRANSFER, TRUE);curl_setopt($ch, CURLOPT_HEADER, FALSE);curl_setopt($ch, CURLOPT_POST, TRUE);curl_setopt($ch, CURLOPT_POSTFIELDS, "{\"user_email\": \"test@smartvault.com\"}");curl_setopt($ch, CURLOPT_HTTPHEADER, array("Authorization: Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==","Content-Type: application/json","Accept: application/json"));$response = curl_exec($ch);curl_close($ch);var_dump($response);
require 'rubygems' if RUBY_VERSION < '1.9'require 'rest_client'values = '{"user_email": "test@smartvault.com"}'headers = {:authorization => 'Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==',:content_type => 'application/json',:accept => 'application/json'}response = RestClient.post 'https://rest.smartvault.com/auto/auth/dtoken/1', values, headersputs response
package mainimport ("bytes""fmt""io/ioutil""net/http")func main() {client := &http.Client{}body := []byte("{\n \"user_email\": \"test@smartvault.com\"\n}")req, _ := http.NewRequest("POST", "https://rest.smartvault.com/auto/auth/dtoken/1", bytes.NewBuffer(body))req.Header.Add("Authorization", "Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==")req.Header.Add("Content-Type", "application/json")req.Header.Add("Accept", "application/json")resp, err := client.Do(req)if err != nil {fmt.Println("Errored when sending request to the server")return}defer resp.Body.Close()resp_body, _ := ioutil.ReadAll(resp.Body)fmt.Println(resp.Status)fmt.Println(string(resp_body))}
//Common testing requirement. If you are consuming an API in a sandbox/test region, uncomment this line of code ONLY for non production uses.//System.Net.ServicePointManager.ServerCertificateValidationCallback = delegate { return true; };//Be sure to run "Install-Package Microsoft.Net.Http" from your nuget command line.using System;using System.Net.Http;var baseAddress = new Uri("https://rest.smartvault.com/");using (var httpClient = new HttpClient{ BaseAddress = baseAddress }){httpClient.DefaultRequestHeaders.TryAddWithoutValidation("authorization", "Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==");httpClient.DefaultRequestHeaders.TryAddWithoutValidation("accept", "application/json");using (var content = new StringContent("{ \"user_email\": \"test@smartvault.com\"}", System.Text.Encoding.Default, "application/json")){using (var response = await httpClient.PostAsync("auto/auth/dtoken/1", content)){string responseData = await response.Content.ReadAsStringAsync();}}}
Dim request = TryCast(System.Net.WebRequest.Create("https://rest.smartvault.com/auto/auth/dtoken/1"), System.Net.HttpWebRequest)request.Method = "POST"request.ContentType = "application/json"request.Headers.Add("authorization", "Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==")request.Accept = "application/json"Using writer = New System.IO.StreamWriter(request.GetRequestStream())Dim byteArray As Byte() = System.Text.Encoding.UTF8.GetBytes("{\""user_email\"": \""test@smartvault.com\""}")request.ContentLength = byteArray.Lengthwriter.Write(byteArray)writer.Close()End UsingDim responseContent As StringUsing response = TryCast(request.GetResponse(), System.Net.HttpWebResponse)Using reader = New System.IO.StreamReader(response.GetResponseStream())responseContent = reader.ReadToEnd()End UsingEnd Using
import groovyx.net.http.RESTClientimport static groovyx.net.http.ContentType.JSONimport groovy.json.JsonSlurperimport groovy.json.JsonOutput@Grab (group = 'org.codehaus.groovy.modules.http-builder', module = 'http-builder', version = '0.5.0')def client = new RESTClient("https://rest.smartvault.com")def emptyHeaders = [:]emptyHeaders."Authorization" = "Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ=="emptyHeaders."Content-Type" = "application/json"emptyHeaders."Accept" = "application/json"def jsonObj = new JsonSlurper().parseText('{"user_email": "test@smartvault.com"}')response = client.post( path : "/auto/auth/dtoken/1",body : jsonObj,headers: emptyHeaders,contentType : JSON )println("Status:" + response.status)if (response.data) {println("Content Type: " + response.contentType)println("Body:\n" + JsonOutput.prettyPrint(JsonOutput.toJson(response.data)))}
NSURL *URL = [NSURL URLWithString:@"https://rest.smartvault.com/auto/auth/dtoken/1"];NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:URL];[request setHTTPMethod:@"POST"];[request setValue:@"Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==" forHTTPHeaderField:@"Authorization"];[request setValue:@"application/json" forHTTPHeaderField:@"Content-Type"];[request setValue:@"application/json" forHTTPHeaderField:@"Accept"];[request setHTTPBody:[@"{\n \"user_email\": \"test@smartvault.com\"\n}" dataUsingEncoding:NSUTF8StringEncoding]];NSURLSession *session = [NSURLSession sharedSession];NSURLSessionDataTask *task = [session dataTaskWithRequest:requestcompletionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {if (error) {// Handle error...return;}if ([response isKindOfClass:[NSHTTPURLResponse class]]) {NSLog(@"Response HTTP Status code: %ld\n", (long)[(NSHTTPURLResponse *)response statusCode]);NSLog(@"Response HTTP Headers:\n%@\n", [(NSHTTPURLResponse *)response allHeaderFields]);}NSString* body = [[NSString alloc] initWithData:data encoding:NSUTF8StringEncoding];NSLog(@"Response Body:\n%@\n", body);}];[task resume];
import Foundation// NOTE: Uncommment following two lines for use in a Playground// import PlaygroundSupport// PlaygroundPage.current.needsIndefiniteExecution = truelet url = URL(string: "https://rest.smartvault.com/auto/auth/dtoken/1")!var request = URLRequest(url: url)request.httpMethod = "POST"request.addValue("Basic dGVzdHVzZXJAc21hcnR2YXVsdC5jb206UTB4Sk1EQUFBQUFBQUFBQlVZRE9MOE82N3oyQjdvVmJLcytWMngybmZHTXgzR2FzY2pNUEp4Y0dGeHZPeWc9PQ==", forHTTPHeaderField: "Authorization")request.addValue("application/json", forHTTPHeaderField: "Content-Type")request.addValue("application/json", forHTTPHeaderField: "Accept")request.httpBody = """"{\n \"user_email\": \"test@smartvault.com\"\n}"""".data(using: .utf8)let task = URLSession.shared.dataTask(with: request) { data, response, error inif let response = response {print(response)if let data = data, let body = String(data: data, encoding: .utf8) {print(body)}} else {print(error ?? "Unknown error")}}task.resume()
Response
Returns the delegation token, its expiracy and the id related to the user used for retrieving it.
Show success object
Will return an error object if using the autonomous authentication and the Authorization header is not specified (access denied) or an error object if the "user_email" of the body is missing.